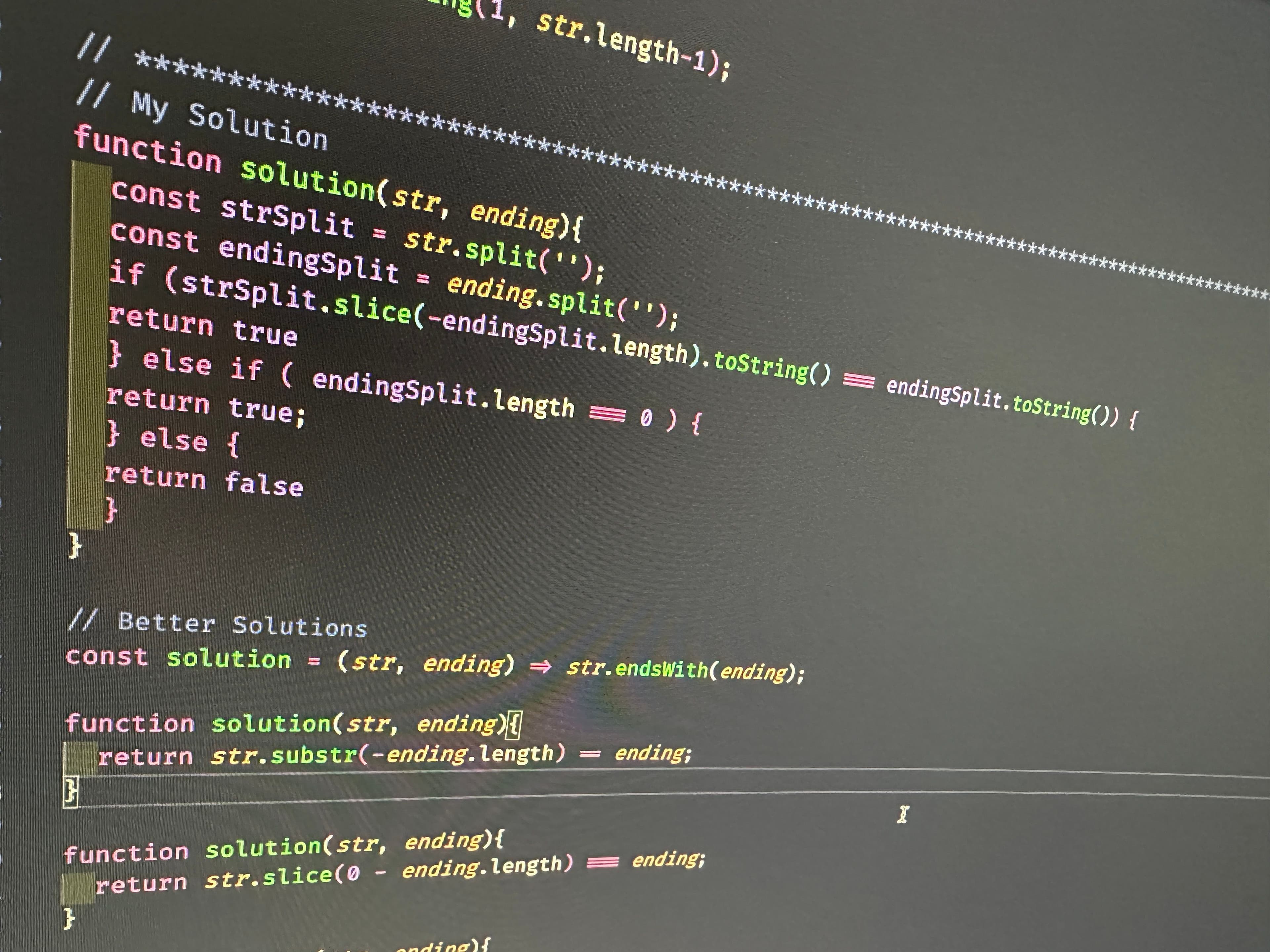
My code sucks.
February 26, 2023

Sam Wynne
Sam Wynne is a tech consultant with a background in restaurant and brewery ownership.
This is the first installment of a series on my return to daily JavaScript Algorithms.
JS Fundamentals
After spending the last year focusing on Front End frameworks like React and Vue, I have gotten away from daily JavaScript Algorithm practice. I have gotten embarrassingly rusty. My first impulse was to do everything I could to hide this from the world… but instead I decided to put myself on blast. Why does my code suck? How could I make it better? Who wrote code that I wish mine looked like? This blog series will focus on that, thanks to https://www.codewars.com.
Why would I embarrass myself like this?
I got the same advice from two different people I respect in ONE week. On the tech side, it was Max DeMarzi who’s blog https://maxdemarzi.com/ is my current go to for Graph Database wisdom. I was watching a Neo4J lecture of his on YouTube and when asked why he blogs, he said that there is always someone that knows more than you and always someone that knows less than you, but most importantly there is always someone that is only ONE DAY behind you. Some small thing you share could really help that person out on their journey.
The other was more general advice for creative types by author Austin Kleon in his book “Show Your Work”. https://austinkleon.com/show-your-work/ It is part of a VERY short trilogy of books that I highly recommend, but I have too much to say about that series to get into it right now.
Algorithm #1
The challenge is to create a function with two parameters, (str, ending) where str is a string and ending is a string of equal or lesser length, that returns true if the original str ends with ending.
My code
function solution(str, ending){ const strSplit = str.split(''); const endingSplit = ending.split(''); if (strSplit.slice(-endingSplit.length).toString() === endingSplit.toString()) { return true } else if ( endingSplit.length === 0 ) { return true; } else { return false } }
Yes, I told you it was ugly. To be fair, I wrote this with the expectation that no one would ever see it and I was simple completing a logic puzzle. Then CodeWars showed me other solutions to the same challenge and I realized I needed to step my game up, and this series was born.
Let’s start with the obvious, that if else chain is as junior as it gets and sticks out like a drunk sports fan with face paint before you even start reading the function. Then I immediately start wasting time by converting the strings to arrays, only to immediately turn them right back to strings. UGH. One of the test cases was actually where ending was an empty string, so I sloppily hacked around that edge case too. Honestly, I can’t really come up with anything nice to say about my solution at all. Ok… I feel better now.
Slightly Better Code
function solution(str, ending){ var l = ending.length; var str = str.slice(-l); return str.match(ending) ? true : false; }
This code is one step better for sure. It doesn’t have the unnecessary string to array and back again, and the ternary operator at the end is way classier than the if/else garbage I let fall out of my monkey brain.
If I may be critical of someone else code that is better than mine, I try my best to avoid var unless there is a rare and unique reason that makes me need to. Next, the var l = ending.length seems entirely unnecessary since l is only used once. Also, I think that .match() is clever, but the next few solutions are a bit more elegant. MDN Docs on .match() : https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/match
Substantially Better Code
function solution(str, ending){ return str.slice(0 - ending.length) === ending; }
function solution(str, ending){ return str.substr(-ending.length) == ending; }
In my option, these two solutions are equivalently better than my code and the previous solution. They take the challenge and just do it in one line. The .slice() is a bit more my style. The .substr() is a hair more elegant but is deprecated/not recommended due to lack of browser support and .substring() is considered the better move here at the time of this writing. .slice() Docs: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/slice .substr() Docs: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/substr .substring() Docs: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/substring
Best Code
const solution = (*str*, *ending*) => *str*.endsWith(*ending*);
Short. Sweet. To the point. Maximizes ES6 features, and even includes a minor refactor of the code presented in the challenge. Just like the last few examples it utilizes methods that return the desired boolean result. Showcases a broad knowledge of JavaScript to solve the problem with the fewest characters possible without sacrificing readability. Just lovely. .endsWith() Docs: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/endsWith
Thanks for reading, I look forward to more of these soon. Cheers.